vJoystick
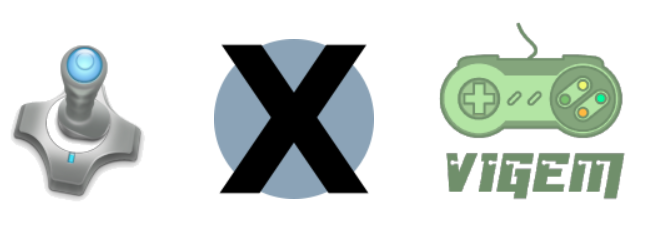
vJoystick
可橋接非遊戲控制器的任何設備與需要遊戲控制器的應用程式的設備驅動程序
http://vjoystick.sourceforge.net/joomla/
Download
https://sourceforge.net/projects/vjoystick/ or https://github.com/shauleiz/vJoy
vJoystick with UCR
- https://home.gamer.com.tw/creationDetail.php?sn=4886070
- https://www.youtube.com/watch?v=SL3mXAHH-Eg&feature=emb_title
Source Code : tajtiattila/vjoy
https://github.com/tajtiattila/vjoy
Include dll
|
|
Variable
Axis values can be:
inc/public.h
|
|
Value in the range 0x1-0x8000
dll/dll.go
|
|
Test vJoy Driver
Before you start, check if the vJoy driver is installed and check that it is what you expected:
dll/dll.go
|
|
Test Interface DLL
dll/proc.go
|
|
dll/dll.go
|
|
vjoy.go
|
|
Test vJoy Virtual Devices
dll/dll.go
|
|
|
|
Check which devices are installed and what their state is it. make sure that the axes, buttons (and POV hat switches) are as expected
vjoy.go
|
|
Acquire the vJoy Device:
Until now you just made inquiries about the system and about the vJoy device status. In order to change the position of the vJoy device you need to Acquire it (if it is not already owned):
|
|
Feed vJoy Device
- Efficient: Collect position data, place the data in a position structure then finally send the data to the device.
- Robust: Reset the device once then send the position data for every control (axis, button,POV) at a time.
Relinquish the vJoy Device:
You must relinquish the device when the driver exits: RelinquishVJD(iInterface);
vjoy.go
|
|
dll/dll.go
|
|
Interface Function Reference:
General driver data
vJoyEnabled
Returns TRUE if vJoy version 2.x is installed and enabled.
|
|
GetvJoyVersion
Return the version number of the installed vJoy. To be used only after vJoyEnabled()
|
|
GetvJoyProductString / GetvJoyManufacturerString / GetvJoySerialNumberString
These functions return an LPTSTR that points to the correct data (Product, Manufacturer or Serial number). To be used only after vJoyEnabled()
|
|
DriverMatch
Returns TRUE if vJoyInterface.dll file version and vJoy Driver version are identical. Otherwise returns FALSE. Optional (You may pass NULL): Output parameter DllVer: If a pointer to WORD is passed then the value of the DLL file version will be written to this parameter (e.g. 0x215). Output parameter DrvVer: If a pointer to WORD is passed then the value of the Driver version will be written to this parameter (e.g. 0x215).
|
|
RegisterRemovalCB
- This function registers a user-defined ConfChangedCB callback fuction that is called everytime a vJoy device is added or removed. Paremeter ConfChangedCB is a pointer to the user-defined callback function. Parameter UserData is a pointer to a user-defined data item. The callback function recieves this pointer as its third parameter. More in section Detecting Changes.
|
|
__cdecl : https://docs.microsoft.com/zh-tw/cpp/cpp/cdecl?view=msvc-170 __declspec : https://docs.microsoft.com/zh-tw/cpp/cpp/declspec?view=msvc-170
Write access to vJoy Device
The following functions access the virtual device by its ID (rID). The value of rID may vary between 1 and 16. There may be more than one virtual device installed on a given system. VJD stands for Virtual Joystick Device.
GetVJDStatus
Returns the status of the specified device The status can be one of the following values:
|
|
|
|
[v2.1.6] isVJDExists
Returns TRUE if the specified device exists (Configured and enabled). Returns FALSE otherwise (Including the following cases: Device does not exist, disabled, driver not installed)
|
|
[v2.1.8] GetOwnerPid
Returns the Process ID (PID) of the process that owns the specified device. If the device is owned by a process, then the function returns a positive integer which is the PID of the owner. Otherwise, the function returns one of the following negative numbers: NO_FILE_EXIST (-13): Usually indicates a FREE device (No owner) NO_DEV_EXIST (-12): Usually indicates a MISSING device BAD_DEV_STAT (-11): Indicates some internal problem
|
|
Write access to vJoy Device - Basic
AcquireVJD
Acquire the specified device. Only a device in state VJD_STAT_FREE can be acquired. If acquisition is successful the function returns TRUE and the device status becomes VJD_STAT_OWN.
|
|
RelinquishVJD
Relinquish the previously acquired specified device. Use only when device is state VJD_STAT_OWN. State becomes VJD_STAT_FREE immediately after this function returns.
|
|
UpdateVJD
Update the position data of the specified device. Use only after device has been successfully acquired. Input parameter is a pointer to structure of type JOYSTICK_POSITION that holds the position data. Returns TRUE if device updated.
|
|
vJoy Device properties
The following functions receive the virtual device ID (rID) and return the relevant data. The value of rID may vary between 1 and 16. There may be more than one virtual device installed on a given system. The return values are meaningful only if the specified device exists VJD stands for Virtual Joystick Device.
GetVJDButtonNumber
If function succeeds, returns the number of buttons in the specified device. Valid values are 0 to 128 If function fails, returns a negative error code: • NO_HANDLE_BY_INDEX • BAD_PREPARSED_DATA • NO_CAPS • BAD_N_BTN_CAPS • BAD_BTN_CAPS • BAD_BTN_RANGE
|
|
GetVJDDiscPovNumber
Returns the number of discrete-type POV hats in the specified device Discrete-type POV Hat values may be North, East, South, West or neutral Valid values are 0 to 4 (from version 2.0.1)
|
|
GetVJDContPovNumber
Returns the number of continuous-type POV hats in the specified device continuous-type POV Hat values may be 0 to 35900 Valid values are 0 to 4 ( from version 2.0.1)
|
|
GetVJDAxisExist
Returns TRUE is the specified axis exists in the specified device Axis values can be:
|
|
|
|
Robust write access to vJoy Devices
The following functions receive the virtual device ID (rID) and return the relevant data. These functions hide the details of the position data structure by allowing you to alter the value of a specific control. The downside of these functions is that you inject the data to the device serially as opposed to function UpdateVJD(). The value of rID may vary between 1 and 16. There may be more than one virtual device installed on a given system.
ResetVJD
Resets all the controls of the specified device to a set of values. These values are hard coded in the interface DLL and are currently set as follows: • Axes X, Y & Z: Middle point. • All other axes: 0. • POV Switches: Neutral (-1) • Buttons: Not Pressed (0).
|
|
ResetAll
Resets all the controls of the all devices to a set of values. See function Reset VJD for details.
|
|
ResetButtons
Resets all buttons (To 0) in the specified device.
|
|
ResetPovs
Resets all POV Switches (To -1) in the specified device.
|
|
SetAxis
Write Value to a given axis defined in the specified VDJ. Value in the range 0x1-0x8000 Axis can be one of the following:
HID_USAGE_X // X Axis HID_USAGE_Y // Y Axis HID_USAGE_Z // Z Axis HID_USAGE_RX // Rx Axis HID_USAGE_RY // Ry Axis HID_USAGE_RZ // Rz Axis HID_USAGE_SL0 // Slider 0 HID_USAGE_SL1 // Slider 1 HID_USAGE_WHL // Wheel
|
|
SetBtn
Write Value (TRUE or FALSE) to a given button defined in the specified VDJ. nBtn can in the range 1-128
|
|
SetDiscPov
Write Value to a given discrete POV defined in the specified VDJ Value can be one of the following: 0: North (or Forwards) 1: East (or Right) 2: South (or backwards) 3: West (or left) -1: Neutral (Nothing pressed) nPov selects the destination POV Switch. It can be 1 to 4
|
|
SetContPov
Write Value to a given continuous POV defined in the specified VDJ Value can be in the range: -1 to 35999. It is measured in units of one-hundredth a degree. -1 means Neutral (Nothing pressed). nPov selects the destination POV Switch. It can be 1 to 4
|
|
FFB Functions
The following functions are used for accessing and manipulating Force Feedback data.
FfbRegisterGenCB
Register a FFB callback function that will be called by the driver every time a FFB data packet arrives. For additional information see Receptor Unit section.
|
|
FfbStart
Enable the FFB mechanism of the specified VDJ Return TRUE on success. Otherwise return FALSE.
|
|
FfbStop
Disable the FFB mechanism of the specified VDJ
|
|
IsDeviceFfb
Return TRUE if specified device supports FFB. Otherwise return FALSE.
|
|
IsDeviceFfbEffect
Return TRUE if specified device supports a specific FFB Effect. Otherwise return FALSE. The FFB Effect is indicated by its Usage. List of effect Usages:
HID_USAGE_CONST (0x26):Usage ET Constant Force Usage HID_USAGE_RAMP (0x27): ET Ramp HID_USAGE_SQUR (0x30): Usage ET Square HID_USAGE_SINE (0x31): Usage ET Sine HID_USAGE_TRNG (0x32): Usage ET Triangle HID_USAGE_STUP (0x33): Usage ET Sawtooth Up HID_USAGE_STDN (0x34): Up Usage ET Sawtooth Down HID_USAGE_SPRNG (0x40): Usage ET Spring HID_USAGE_DMPR (0x41): Usage ET Damper HID_USAGE_INRT (0x42): Usage ET Inertia HID_USAGE_FRIC (0x43): Usage ET Friction
|
|
FFB Helper Functions
Ffb_h_DeviceID
Get the origin of the FFB data packet. If valid device ID was found then returns ERROR_SUCCESS and sets the ID (Range 1-15) in DeviceID. If Packet is NULL then returns ERROR_INVALID_PARAMETER. DeviceID is undefined. If Packet is malformed or Device ID is out of range then returns ERROR_INVALID_DATA. DeviceID is undefined.
|
|
Ffb_h_Type
Get the type of the FFB data packet. Type may be one of the following: // Write PT_EFFREP // Usage Set Effect Report PT_ENVREP // Usage Set Envelope Report PT_CONDREP // Usage Set Condition Report PT_PRIDREP // Usage Set Periodic Report PT_CONSTREP // Usage Set Constant Force Report PT_RAMPREP // Usage Set Ramp Force Report PT_CSTMREP // Usage Custom Force Data Report PT_SMPLREP // Usage Download Force Sample PT_EFOPREP // Usage Effect Operation Report PT_BLKFRREP // Usage PID Block Free Report PT_CTRLREP // Usage PID Device Control PT_GAINREP // Usage Device Gain Report PT_SETCREP // Usage Set Custom Force Report
// Feature PT_NEWEFREP // Usage Create New Effect Report PT_BLKLDREP // Usage Block Load Report PT_POOLREP // Usage PID Pool Report
|
|
If valid Type was found then returns ERROR_SUCCESS and sets Type. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Feature is undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Feature is undefined.
Ffb_h_Packet
Extract the raw FFB data packet and the command type (Write/Set Feature). If valid Packet was found then returns ERROR_SUCCESS and - Sets Type to IOCTRL value (Expected values are IOCTL_HID_WRITE_REPORT and IOCTL_HID_SET_FEATURE). Sets DataSize to the size (in bytes) of the payload data (FFB_DATA.data ). Sets Data to the payload data (FFB_DATA.data ) - this is an array of bytes. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_EBI
Get the Effect Block Index If valid Packet was found then returns ERROR_SUCCESS and sets Index to the value of Effect Block Index (if applicable). Expected value is ‘1’. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed or does not contain an Effect Block Index then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Const
Get parameters of an Effect of type Constant (PT_EFFREP) Effect structure (FFB_EFF_CONST) definition:
|
|
If Constant Effect Packet was found then returns ERROR_SUCCESS and fills structure Effect If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Ramp
Get parameters of an Effect of type Ramp (PT_RAMPREP) Effect structure (FFB_EFF_RAMP) definition:
|
|
If Ramp effect Packet was found then returns ERROR_SUCCESS and fills structure Effect. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_EffOp
Get parameters of an Effect of type Operation (PT_EFOPREP) that describe the effect operation (Start/Solo/Stop) and loop count. Effect structure (FFB_EFF_OP) definition:
|
|
If Operation Effect Packet was found then returns ERROR_SUCCESS and fills structure Operation- this structure holds Effect Block Index, Operation(Start, Start Solo, Stop) and Loop Count. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Period
Get parameters of an Effect of type Periodic (PT_PRIDREP) that describe the periodic attribute of an effect. Effect structure (FFB_EFF_PERIOD) definition:
|
|
If Periodic Packet was found then returns ERROR_SUCCESS and fills structure Effect – this structure holds Effect Block Index, Magnitude, Offset, Phase and period. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Cond
Get parameters of an Effect of type Conditional (PT_CONDREP). Effect structure (FFB_EFF_COND) definition:
|
|
If Condition Packet was found then returns ERROR_SUCCESS and fills structure Condition - this structure holds Effect Block Index, Direction (X/Y), Centre Point Offset, Dead Band and other conditions. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Envlp
Get parameters of an Effect of type Envelope (PT_ENVREP). Effect structure (FFB_EFF_ENVLP) definition:
|
|
If Envelope Packet was found then returns ERROR_SUCCESS and fills structure Envelope If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_EffNew
Get the type of the next effect. Parameter Effect can get one of the following values:
ET_NONE = 0 // No Force ET_CONST = 1 // Constant Force ET_RAMP = 2 // Ramp ET_SQR = 3 // Square ET_SINE = 4 // Sine ET_TRNGL = 5 // Triangle ET_STUP = 6 // Sawtooth Up ET_STDN = 7 // Sawtooth Down ET_SPRNG = 8 // Spring ET_DMPR = 9 // Damper ET_INRT = 10 // Inertia ET_FRCTN = 11 // Friction ET_CSTM = 12 // Custom Force Data
If valid Packet was found then returns ERROR_SUCCESS and sets the new Effect type If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_Eff_Constant
Get parameters of an Effect of type Constant ( ). If Constant Packet was found then returns ERROR_SUCCESS and fills structure ConstantEffect If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
|
|
Ffb_h_DevCtrl
Get device-wide control instructions. Control can get one of the following values:
CTRL_ENACT = 1 // Enable all device actuators. CTRL_DISACT = 2 // Disable all the device actuators. CTRL_STOPALL = 3 // Stop All Effects Issues a stop on every running effect. CTRL_DEVRST = 4 // Device Reset. Clears any device paused condition, enables all actuators and clears all effects from memory. CTRL_DEVPAUSE = 5 // Device Pause. All effects on the device are paused at the current.time step. CTRL_DEVCONT = 6 // Device Continue. All effects that running when the device was paused are restarted from their last time step.
|
|
Ffb_h_DevGain
Get device Global gain in parameter Gain. If valid Packet was found then returns ERROR_SUCCESS and gets the device global gain. If Packet is NULL then returns ERROR_INVALID_PARAMETER. Output parameters are undefined. If Packet is malformed then returns ERROR_INVALID_DATA. Output parameters are undefined.
XOutput
將遊戲控制器的輸入轉換爲 Xbox 360 的信號輸入 https://github.com/csutorasa/XOutput
ViGEmBus
XOutput需要的一個Windows遊戲控制器模擬器 https://github.com/ViGEm/ViGEmBus